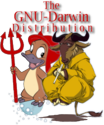
|
RenderState base class.
Inheritance:
Public Fields-
bool _stageDrawnThisFrame
-
DependencyList _dependencyList
-
osg::ref_ptr<osg::Viewport> _viewport
-
GLbitfield _clearMask
-
osg::ref_ptr<osg::ColorMask> _colorMask
-
osg::Vec4 _clearColor
-
osg::Vec4 _clearAccum
-
double _clearDepth
-
int _clearStencil
-
mutable osg::ref_ptr<RenderStageLighting> _renderStageLighting
Public Methods-
RenderStage(SortMode mode=SORT_BY_STATE)
-
RenderStage(const RenderStage& rhs, const osg::CopyOp& copyop=osg::CopyOp::SHALLOW_COPY)
-
virtual osg::Object* cloneType() const
-
virtual osg::Object* clone(const osg::CopyOp& copyop) const
-
virtual bool isSameKindAs(const osg::Object* obj) const
-
virtual const char* className() const
-
virtual void reset()
-
void setViewport(osg::Viewport* viewport)
- Set the viewport
-
const osg::Viewport* getViewport() const
- Get the const viewport.
-
osg::Viewport* getViewport()
- Get the viewport.
-
void setClearMask(GLbitfield mask)
- Set the clear mask used in glClear().
-
GLbitfield getClearMask() const
- Get the clear mask
-
void setColorMask(osg::ColorMask* cm)
-
osg::ColorMask* getColorMask()
-
const osg::ColorMask* getColorMask() const
-
void setClearColor(const osg::Vec4& color)
- Set the clear color used in glClearColor().
-
const osg::Vec4& getClearColor() const
- Get the clear color
-
void setClearAccum(const osg::Vec4& color)
- Set the clear accum used in glClearAccum().
-
const osg::Vec4& getClearAccum() const
- Get the clear accum
-
void setClearDepth(double depth)
- Set the clear depth used in glClearDepth().
-
double getClearDepth() const
- Get the clear depth
-
void setClearStencil(int stencil)
- Set the clear stencil value used in glClearStencil().
-
int getClearStencil() const
- Get the clear color
-
void setRenderStageLighting(RenderStageLighting* rsl)
-
RenderStageLighting* getRenderStageLighting() const
-
virtual void addPositionedAttribute(osg::RefMatrix* matrix, const osg::StateAttribute* attr)
-
virtual void drawPreRenderStages(osg::State& state, RenderLeaf*& previous)
-
virtual void draw(osg::State& state, RenderLeaf*& previous)
-
virtual void drawImplementation(osg::State& state, RenderLeaf*& previous)
-
void addToDependencyList(RenderStage* rs)
-
bool getStats(osg::Statistics* primStats)
- extract stats for current draw list.
Public Members-
typedef std::vector< osg::ref_ptr<RenderStage> > DependencyList
Protected Methods-
virtual ~RenderStage()
Public Fields-
int _binNum
-
RenderBin* _parent
-
RenderStage* _stage
-
RenderBinList _bins
-
RenderGraphList _renderGraphList
-
RenderLeafList _renderLeafList
-
SortMode _sortMode
-
osg::ref_ptr<SortCallback> _sortCallback
-
osg::ref_ptr<DrawCallback> _drawCallback
-
static RenderBinPrototypeList s_renderBinPrototypeList
Public Methods-
static RenderBin* createRenderBin(const std::string& binName)
-
static RenderBin* getRenderBinPrototype(const std::string& binName)
-
static void addRenderBinPrototype(const std::string& binName, RenderBin* proto)
-
static void removeRenderBinPrototype(RenderBin* proto)
-
virtual const char* libraryName() const
-
RenderBin* find_or_insert(int binNum, const std::string& binName)
-
void addRenderGraph(RenderGraph* rg)
-
void sort()
-
virtual void sortImplementation()
-
void setSortMode(SortMode mode)
-
SortMode getSortMode() const
-
virtual void sortByState()
-
virtual void sortFrontToBack()
-
virtual void sortBackToFront()
-
void setSortCallback(SortCallback* sortCallback)
-
SortCallback* getSortCallback()
-
const SortCallback* getSortCallback() const
-
void setDrawCallback(DrawCallback* drawCallback)
-
DrawCallback* getDrawCallback()
-
const DrawCallback* getDrawCallback() const
-
void getPrims(osg::Statistics* primStats)
-
bool getPrims(osg::Statistics* primStats, int nbin)
-
void copyLeavesFromRenderGraphListToRenderLeafList()
Public Members-
typedef std::vector<RenderLeaf*> RenderLeafList
-
typedef std::vector<RenderGraph*> RenderGraphList
-
typedef std::map< int, osg::ref_ptr<RenderBin> > RenderBinList
-
enum SortMode
-
struct SortCallback: public osg::Referenced
-
struct DrawCallback: public osg::Referenced
-
typedef std::map< std::string, osg::ref_ptr<RenderBin> > RenderBinPrototypeList
Documentation
RenderState base class. Used for encapsulate a complete stage in
rendering - setting up of viewport, the projection and model
matrices and rendering the RenderBin's enclosed with this RenderStage.
RenderStage also has a dependency list of other RenderStages, each
of which must be called before the rendering of this stage. These
'pre' rendering stages are used for advanced rendering techniques
like multistage pixel shading or impostors.
RenderStage(SortMode mode=SORT_BY_STATE)
RenderStage(const RenderStage& rhs, const osg::CopyOp& copyop=osg::CopyOp::SHALLOW_COPY)
virtual osg::Object* cloneType() const
virtual osg::Object* clone(const osg::CopyOp& copyop) const
virtual bool isSameKindAs(const osg::Object* obj) const
virtual const char* className() const
virtual void reset()
void setViewport(osg::Viewport* viewport)
- Set the viewport
const osg::Viewport* getViewport() const
- Get the const viewport.
osg::Viewport* getViewport()
- Get the viewport.
void setClearMask(GLbitfield mask)
- Set the clear mask used in glClear().
Defaults to GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT.
GLbitfield getClearMask() const
- Get the clear mask
void setColorMask(osg::ColorMask* cm)
osg::ColorMask* getColorMask()
const osg::ColorMask* getColorMask() const
void setClearColor(const osg::Vec4& color)
- Set the clear color used in glClearColor().
glClearColor is only called if mask & GL_COLOR_BUFFER_BIT is true
const osg::Vec4& getClearColor() const
- Get the clear color
void setClearAccum(const osg::Vec4& color)
- Set the clear accum used in glClearAccum().
glClearAcumm is only called if mask & GL_ACCUM_BUFFER_BIT is true
const osg::Vec4& getClearAccum() const
- Get the clear accum
void setClearDepth(double depth)
- Set the clear depth used in glClearDepth(). Defaults to 1.0
glClearDepth is only called if mask & GL_DEPTH_BUFFER_BIT is true
double getClearDepth() const
- Get the clear depth
void setClearStencil(int stencil)
- Set the clear stencil value used in glClearStencil(). Defaults to 1.0
glClearStencil is only called if mask & GL_STENCIL_BUFFER_BIT is true
int getClearStencil() const
- Get the clear color
void setRenderStageLighting(RenderStageLighting* rsl)
RenderStageLighting* getRenderStageLighting() const
virtual void addPositionedAttribute(osg::RefMatrix* matrix, const osg::StateAttribute* attr)
virtual void drawPreRenderStages(osg::State& state, RenderLeaf*& previous)
virtual void draw(osg::State& state, RenderLeaf*& previous)
virtual void drawImplementation(osg::State& state, RenderLeaf*& previous)
void addToDependencyList(RenderStage* rs)
bool getStats(osg::Statistics* primStats)
- extract stats for current draw list.
typedef std::vector< osg::ref_ptr<RenderStage> > DependencyList
bool _stageDrawnThisFrame
DependencyList _dependencyList
osg::ref_ptr<osg::Viewport> _viewport
GLbitfield _clearMask
osg::ref_ptr<osg::ColorMask> _colorMask
osg::Vec4 _clearColor
osg::Vec4 _clearAccum
double _clearDepth
int _clearStencil
mutable osg::ref_ptr<RenderStageLighting> _renderStageLighting
virtual ~RenderStage()
- Direct child classes:
- RenderToTextureStage
Alphabetic index HTML hierarchy of classes or Java
This page was generated with the help of DOC++.
|