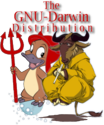
|
# Copyright (c) 2001 David Muse
# See the file COPYING for more information
class Driver
connect(dbname, user, auth, attr)
dbname should have the format:
"DBI:SQLRelay:host=host;port=port;socket=socket;"
Opens a connection to the sqlrelay server and
authenticates with user and auth. attr is
currently ignored.
class Database
disconnect()
Ends the current session.
prepareQuery(query)
Prepare to execute query. Returns a Statement.
ping()
Returns true if the database is up and false
if it's down.
commit()
Issues a commit.
Raises a DBI::ProgrammingError if it failed.
rollback()
Issues a rollback.
Raises a DBI::ProgrammingError if it failed.
[]=(attr,value)
Stores "value" associated with key "attr".
sqlrelay_debug=true/false will toggle
debugging and AutoCommit=true/false will
toggle autocommit.
class Statement
bind_param(param, value, attribs)
Binds value to param defined earlier in the
prepare call. attribs is currently unused.
execute()
Send a SQL query to the server and
returns the number of rows returned.
Raises a DBI::ProgrammingError if it failed.
finish()
Invalidates the statement.
fetch()
Returns one row of the result set.
column_info()
Returns an array of hashes.
Each hash contains a 'name', 'type_name'
and 'precision' key.
rows()
Returns the number of rows in the current result set.
fetch_scroll(direction, offset=1)
Returns one row of the result set.
Which row depends on direction and offset.
For DBI::SQL_FETCH_NEXT the next row is returned.
For DBI::SQL_FETCH_PRIOR the prior row is returned.
For DBI::SQL_FETCH_FIRST the first row is returned.
For DBI::SQL_FETCH_LAST the last row is returned.
For DBI::SQL_FETCH_ABSOLUTE the "offset" row is returned.
For DBI::SQL_FETCH_RELATIVE the row "offset"
rows from the current row is returned.
fetch_many(cnt)
Returns the next "cnt" rows rest of the result set.
fetch_all()
Returns the rest of the result set.
|