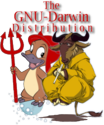
|
SWIG/Examples/ruby/class/
Wrapping a simple C++ class
$Header: /cvs/projects/SWIG/Examples/ruby/class/index.html,v 1.1.4.1 2001/08/30 10:48:04 cheetah Exp $
This example illustrates C++ class wrapping performed by SWIG.
C++ classes are simply transformed into Ruby classes that provide methods to
access class members.
The C++ Code
Suppose you have some C++ classes described by the following (and admittedly lame)
header file:
/* File : example.h */
class Shape {
public:
Shape() {
nshapes++;
}
virtual ~Shape() {
nshapes--;
};
double x, y;
void move(double dx, double dy);
virtual double area() = 0;
virtual double perimeter() = 0;
static int nshapes;
};
class Circle : public Shape {
private:
double radius;
public:
Circle(double r) : radius(r) { };
virtual double area();
virtual double perimeter();
};
class Square : public Shape {
private:
double width;
public:
Square(double w) : width(w) { };
virtual double area();
virtual double perimeter();
};
The SWIG interface
A simple SWIG interface for this can be built by simply grabbing the header file
like this:
/* File : example.i */
%module example
%{
#include "example.h"
%}
/* Let's just grab the original header file here */
%include "example.h"
Note: when creating a C++ extension, you must run SWIG with the -c++ option like this:
% swig -c++ -ruby example.i
A sample Ruby script
Click here to see a script that calls the C++ functions from Ruby.
Key points
- To create a new object, you call a constructor like this:
c = Example::Circle.new(10)
- To access member data, a pair of accessor methods are used.
For example:
c.x = 15 # Set member data
x = c.x # Get member data
- To invoke a member function, you simply do this
print "The area is ", c.area, "\n"
- When a instance of Ruby level wrapper class is garbage collected by
Ruby interpreter, the corresponding C++ destructor is automatically invoked.
(Note: destructors are currently not inherited. This might change later.
Until then, use -make_default).
- Static member variables are wrapped as Ruby class accessor methods.
For example:
n = Shape.nshapes # Get a static data member
Shapes.nshapes = 13 # Set a static data member
General Comments
- Ruby module of SWIG differs from other language modules in wrapping C++
interfaces. They provides lower-level interfaces and optional higher-level
interfaces know as shadow classes. Ruby module needs no such redundancy
due to Ruby's sophisticated extension API.
- SWIG *does* know how to properly perform upcasting of objects in
an inheritance hierarchy except for multiple inheritance.
- A wide variety of C++ features are not currently supported by SWIG. Here is the
short and incomplete list:
- Overloaded methods and functions. SWIG wrappers don't know how to resolve name
conflicts so you must give an alternative name to any overloaded method name using the
%name directive like this:
void foo(int a);
%name(foo2) void foo(double a, double b);
- Overloaded operators. Not supported at all. The only workaround for this is
to write a helper function. For example:
%inline %{
Vector *vector_add(Vector *a, Vector *b) {
... whatever ...
}
%}
- Namespaces. Not supported at all. Won't be supported until SWIG2.0 (if at all).
- Dave's snide remark: Like a large bottle of strong Tequilla, it's better to
use C++ in moderation.
|