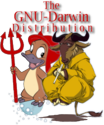
|
PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
GSL_Matrix#LU_decomp
-
This method factorizes the square matrix into the LU decomposition.
This method returns an array of three elements. The first is the matrix of the LU
decomposition, the second is a GSL_permutation object. The third is the sign of the
permutation.
GSL_Matrix#LU_solve(perm, b)
-
This method solves the system A x = b using the LU decomposition of A
given by LU_decomp and the permutation perm. The returned value is a
GSL_vector object which contains the solution vector x. The following is an
example to solve a linear system
A x = b, b = (1, 2, 3, 4)
with LU_decomp and LU_solve ,
ex.)
(require 'narray') <--- if Ruby/GSL is compiled with --with-narray flag
require 'gsl'
m = GSL_Matrix.new([0.18, 0.60, 0.57, 0.96], [0.41, 0.24, 0.99, 0.58],
[0.14, 0.30, 0.97, 0.66], [0.51, 0.13, 0.19, 0.85])
lu, p = m.LU_decomp <- m is decomposed into lu
b = [1, 2, 3, 4]
x = lu.LU_solve(p, b.to_v) <- "b" must be given as a GSL_Vector
p x.to_a <- The solution is returned by a vector.
GSL_Matrix#LU_invert(perm)
-
This method computes and returns the inverse of a matrix from its LU decomposition.
This requires the permutation perm given by LU_decomp.
ex.)
lu, p, signum = m.LU_decomp
inv = lu.LU_invert(p)
inv.print
GSL_Matrix#LU_det(sugnum)
-
This method computes and returns the determinant of a matrix from its LU
decomposition. This requires the value of signum given by LU_decomp.
ex.)
lu, p, signum = m.LU_decomp
p lu.LU_det(signum)
GSL_Matrix#SV_decomp
-
This function factorizes the M-by-N matrix into the singular value decomposition
A = U S V^T (A: reciever). This method returns an array of three elements. The
first is a GSL_Matrix object of a matrix U. The second is the matrix V, and the
last is a GSL_vector which corresponds to S.
SV_solve
-
This method solves a linear system using the SV decomposition.
ex.)
m = GSL_Matrix.new([1, 2, 3], [6, 5, 4], [7, 8, 1])
U, V, S = m.SV_decomp
x = gsl_linalg_SV_solve(U, V, S, [2, 3, 4].to_v)
x.print
GSL_Matrix#SVD_solve(b)
-
This method computes the SV decomposition of the reciever matrix, and solve
the linear system with a given vector b.
ex.)
m = GSL_Matrix([1, 2, 3], [6, 5, 4], [7, 8, 1])
b = [2, 3, 4].to_v
x = m.SVD_solve(b)
x.print
|